On Friday, 13 September 2024 at 14:18:28 UTC, ryuukk_ wrote:
> This should be allowed, another DIP idea, if anyone still care about improving the language
I care but is there not enough development? I claim that D is a better language than C pluck pluck :)
Isn't the following enough for you?
import std.typecons;
struct Vector3i { uint x, y, z; }
void main()
{
//Vector3i vector = {z: 3, x: 1, y: 2};/*
auto vector = Vector3i(
z: 3,
x: 1,
y: 2
);//*/
// ...
On Monday, 21 October 2024 at 14:02:20 UTC, ryuukk_ wrote:
> https://x.com/SebAaltonen/status/1848311998376738892/
I took implemented the code written by the people you modeled without getting lazy:
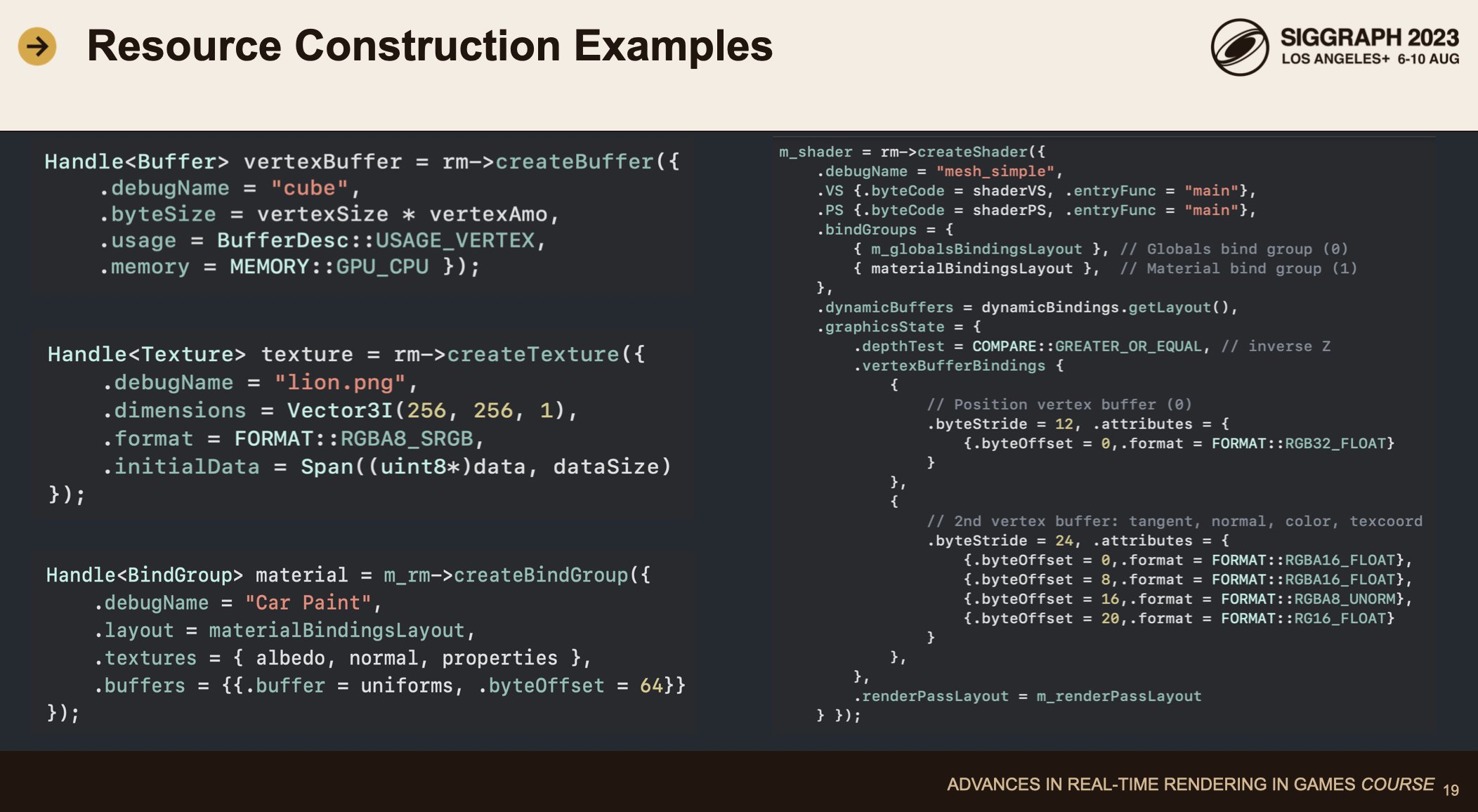
Is the following still not enough? Same code:
// ...
ResourceMaker rm;
auto data = new ubyte[vertexSize];
auto vertexBuffer = rm.createBuffer(
debugName: "cube",
byteSize: vertexSize * vertexAmo,
usage: Usage.vertex,
memory: Memory.GPU_CPU
);
auto texture = rm.createTexture(
debugName: "lion.png",
dimensions: Vector3i(256, 256, 1),
format: Format.RGBA8_SRGB,
initialData: cast(ubyte[])data[0..dataSize]
);
auto uniforms = vertexBuffer;
auto material = BindGroup(
debugName: "Car Paint",
layout: "materialBindingsLayout",
textures: ["albedo", "normal", "properties"],
buffers: [tuple!("buffer", "byteOffset")(uniforms, 64)]
);
}
enum : uint
{
dataSize = 128,
vertexSize = 1024,
vertexAmo = 8,
}
enum Format { RGBA8_SRGB, RGBA16_FLOAT }
enum Usage { vertex, uniform }
enum Memory { CPU, GPU_CPU }
struct BufferDesc
{
string debugName = null;
uint byteSize;
Usage usage = Usage.uniform;
Memory memory = Memory.CPU;
ubyte[] initialData;
}
struct BindGroup
{
string debugName = null;
string layout;
string[] textures;
Tuple!(BufferDesc, "buffer", int, "byteOffset")[] buffers;
}
struct Texture
{
string debugName = null;
Vector3i dimensions;
Format format = Format.RGBA8_SRGB;
ubyte[] initialData;
}
struct ResourceMaker
{
auto createBuffer(uint byteSize, string debugName, Memory memory, Usage usage, ubyte[] initialData = [])
=> BufferDesc(debugName, byteSize, usage, memory, initialData);
auto createTexture(string debugName, Vector3i dimensions, Format format, ubyte[] initialData = [])
=> Texture(debugName, dimensions, format, initialData);
}
SDB@79